I've been working on this game in my free time with a few friends. An early build of it can be found on my 'Projects' page. I'm the Lead (well the only) Programmer on the team and one of the things we wanted was a dynamic camera that smoothly followed the player instead of just snapping to his position. So I got the camera to follow the player after a slight delay which looked nice except in certain situations (see image below).
After that I thought of different ways to add more functionality to the camera to better show the player's surroundings. The best way that I thought of was to have the camera boost it's position in a certain direction based on the direction that the player was traveling.
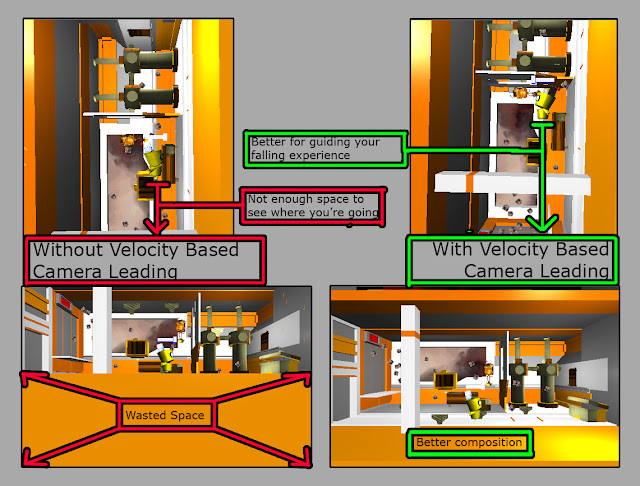
Check out the code
//Script by Devin Curry //www.Devination.com using UnityEngine; using System.Collections; public class CameraFollow : MonoBehaviour { private Transform player = null; public float cameraLag = 0.3f; //needed for Vector3 SmoothDamp private Vector3 velocity = Vector3.zero; //buckets for holding camera and player postions private float cameraZ = 0.0f; private Vector3 newCameraPos; private Vector3 playerv3; //used for boosting the camera based on player's velocity public float boostY = 1.0f; //stored value for camera lead distance private float boostedY = 0.0f; //fluctuates, uses boostY as multiplier with player's velocity void Start() { //finds the player's transform component player = GameObject.FindGameObjectWithTag("Player").transform; cameraZ = this.transform.position.z; //caches initial camera z position } void Update () { playerv3 = player.transform.position; //finds player's current position //checks the player's current velocity Debug.Log(player.rigidbody.velocity); //sets a dead zone to not affect the camera if(player.rigidbody.velocity.y <= -1.5) { //takes the y component of the players velocity and uses it to boost the camera in the direction we want boostedY = player.rigidbody.velocity.normalized.y * boostY; } else if(player.rigidbody.velocity.y > -1.5) { //if the player is not moving, camera will boost itself up by the default amount boostedY = boostY; } //appends camera's Z position to player's X and Y positions newCameraPos = new Vector3 (playerv3.x, (playerv3.y) + boostedY, cameraZ); //assigns new position to camera with a slight camera lag this.transform.position = Vector3.SmoothDamp(this.transform.position, newCameraPos, ref velocity, cameraLag); } }